Python Course aimed at Engineers and Data Scientists
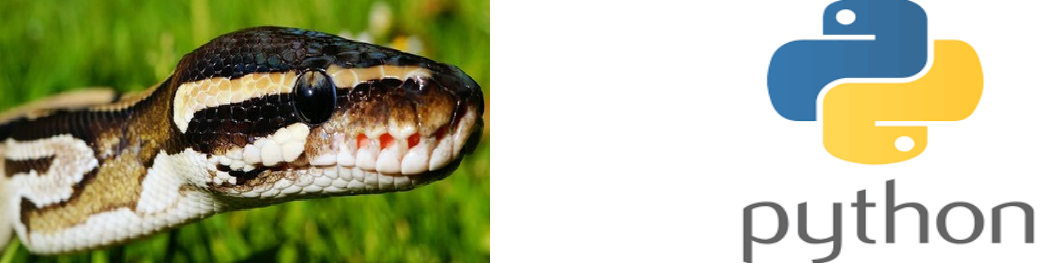
The Python course is intended for people new to Python.
It consists of about 60% exercises with a trainer per 1 to 9 participants helping
individually. At the end of the course participants will have a solid working
knowledge of python and will understand its subtleties. The topics covered are:
1) Python introduction
- Comparison of Python to Java, C, C++.
- Main differences between Python versions 2 and 3.
- Python program and interactive modes.
2) Types, lists, variables, control flow
- Integers, floats, strings and operators on them.
- Lists: construction, usage, main methods.
- Tuples: construction, usage, differences to lists.
- Slicing
- Dictionaries: construction, usage, main methods.
- Comparing lists, tuples, dictionaries with respect to same content versus to same reference.
- Variables
- Loops: for-loop, while-loop, break, continue.
- If-construct.
- Accessing command line parameters.
3) Functions
- Basic form of functions.
- Optional arguments.
- Variable number of arguments.
- Variable number of keyword arguments.
- Variable number of ordinary and keyword arguments.
- Functions as arguments.
- Lambda functions.
- Internal functions.
- Generator functions.
- Documentation of functions and documentation tools.
4) Modules and packages
- Definition / analogy.
- File relative import versus absolute import.
- The __init__.py file.
5) Classes and inheritance
- Class definition
- Class constructor
- Class methods
- Adding / deleting attributes dynamically. Check attribute existence.
- Attributes tied to the class intead of to an instance.
- Deriving a class.
- Private attributes.
- Magic methods __str__(), __repr__(), __call__(), __equals__()
6) Exceptions
- Try-catch construct.
- Reraise an exception.
- Catching a specific exception.
- Define your own Exception.
- Exception class hierarchy is important. Traps.
- Assert / AssertionError
7) Standard Library, Matplotlib, regular expressions
- copy / deepcopy
- join, split, strip Strings
- format strings
- Reading and writing files.
- Modules datetime and date.
- with-construct.
- Serialization of objects.
- System functions. Calling other programs.
- The module os for interactions with the operating system.
- Creating visualizations with Matplotlib.
- The module re: Working with regular expressions in Python.
8) Data bases
- Establishing a connection from a Python program.
- Executing SQL-commands that modify the data basis.
- Obtaining data from a data basis.
9) Web-Services
- Programming the client side with the module requests.
- Programming the server side with Flask.
Each of the listed topics has one or more exercise units. The course duration is 5 days.
On request, this course can be combined with the other courses or shortened with a duration between 2 and 5 days. If you are interested in this course, please send us a message, since we plan courses dynamically on demand.
(Price list).